Other JavaScript Files
These are normally used for Angular Services & Directives
In Creator, you have the ability to create JavaScript files which will be automatically included in your app. Generally, these will be used to define Angular modules that create services, directives, or to configure your app.
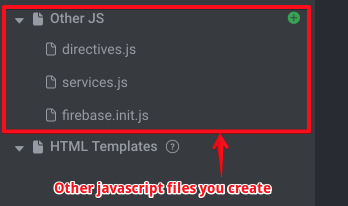
Here are some examples of what you might see in a typical app:
1. Writing Services and Directives
Services are commonly used to serve as a piece of re-usable code that persists data for the life of your app, and has re-usable functions that you can use throughout the rest of your app JavaScript.
For example, if you store some data on a controller $scope variable, that data will be destroyed as soon as you navigate to another view in your app. If you would like some data to persist, it is good practice to use a service for this reason.
Or, if your app relies on an external API to store and retrieve data then you can use a service to provide a re-usable set of functions to communicate with that API. This way you don't have to replicate code across multiple controllers.
For a great example of writing a service, see our example of how to create an HTTP API.
Directives allow you to extend the functionality of HTML elements. You can extend the functionality, or essentially create your own types of HTML elements that are dynamic and interactive. To learn more about directives, see the official Angular docs.
2. Using .config() and .run()
Any code you use in a .config() block will be the first code that runs in your Angular app. An example would be overriding default back button text using $ionicConfigProvider:
// This code would go in the JS file you create in Creator
angular.module('app.config', [])
// remember to add "app.config" to your angular modules in Code Settings
.config(function($ionicConfigProvider) {
$ionicConfigProvider.backButton.text('Go Back').icon('ion-chevron-left');
});
Remember
In this example, you would need to remember to add "app.config" in your root Angular modules. This can be done in the Code Settings section of Creator (accessible via the top bar of the code editor).
Any code in a .run() block will be executed next. An example would be your initial configuration of Firebase:
angular.module('firebaseConfig', ['firebase'])
.run(function(){
// Initialize Firebase
var config = {
apiKey: "MyAPIKey",
authDomain: "something.firebaseapp.com",
databaseURL: "https://something.firebaseio.com",
storageBucket: "something.appspot.com",
};
firebase.initializeApp(config);
})
Once again, in this example you would also need to add "firebaseConfig" to your Angular Modules in Code Settings.
Updated less than a minute ago